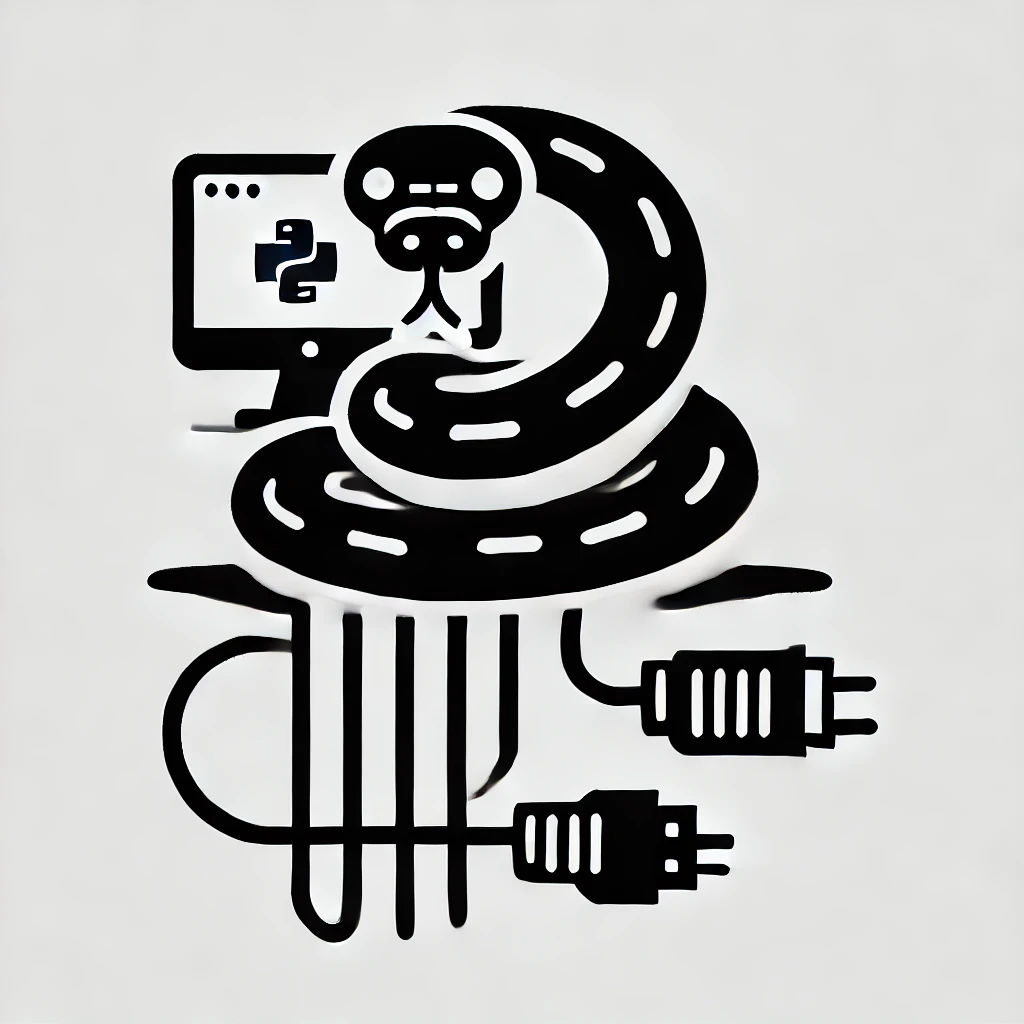
Empower your IT skills with these powerful yet Useful Python scripts
Why Python for IT Apprentices?
Python is a versatile language that can automate many IT tasks, often surpassing the capabilities of built-in Windows commands. These scripts offer powerful functionality that typically requires paid tools, making them invaluable for IT apprentices.
Useful Python Scripts for IT Tasks
1. Active Directory User Creation
Automate the creation of Active Directory users from a CSV file:
import csv
import subprocess
def create_ad_user(username, password, first_name, last_name):
cmd = f'New-ADUser -Name "{username}" -GivenName "{first_name}" -Surname "{last_name}" -UserPrincipalName "{username}@domain.com" -SamAccountName "{username}" -AccountPassword (ConvertTo-SecureString "{password}" -AsPlainText -Force) -Enabled $true'
subprocess.run(["powershell", "-Command", cmd], check=True)
with open('users.csv', 'r') as file:
reader = csv.DictReader(file)
for row in reader:
create_ad_user(row['username'], row['password'], row['first_name'], row['last_name'])
2. Windows Update Management
Install Windows updates automatically:
import subprocess
def install_windows_updates():
cmd = 'powershell.exe Install-WindowsUpdate -AcceptAll -AutoReboot'
subprocess.run(cmd, shell=True, check=True)
install_windows_updates()
3. Network Monitoring
Monitor network traffic using the psutil
library:
import psutil
def network_stats():
net_io = psutil.net_io_counters()
print(f"Bytes Sent: {net_io.bytes_sent}")
print(f"Bytes Received: {net_io.bytes_recv}")
network_stats()
4. Simple Vulnerability Scanner
Scan specified ports on a given IP address:
import socket
def scan_port(ip, port):
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
sock.settimeout(1)
result = sock.connect_ex((ip, port))
if result == 0:
print(f"Port {port} is open on {ip}")
else:
print(f"Port {port} is closed on {ip}")
sock.close()
target_ip = '192.168.1.1'
ports = [22, 80, 443, 8080]
for port in ports:
scan_port(target_ip, port)
5. Password Hashing
Hash passwords using SHA-256:
import hashlib
def hash_password(password):
hashed = hashlib.sha256(password.encode()).hexdigest()
return hashed
password = "your_password"
hashed_password = hash_password(password)
print(f"Hashed Password: {hashed_password}")
Advanced Python Scripts for IT Professionals
1. Wi-Fi Analysis Tool
Analyze nearby Wi-Fi networks (requires admin privileges):
import subprocess
import re
def get_wifi_networks():
output = subprocess.check_output(["netsh", "wlan", "show", "network"])
output = output.decode("ascii")
networks = re.findall("(?:Profile\s*:\s)(.*)", output)
return networks
print("Available Wi-Fi networks:")
for network in get_wifi_networks():
print(network)
2. Advanced Active Directory Management
Retrieve and modify user group memberships:
import subprocess
def get_user_groups(username):
cmd = f'Get-ADUser -Identity {username} -Properties MemberOf | Select-Object -ExpandProperty MemberOf'
result = subprocess.run(["powershell", "-Command", cmd], capture_output=True, text=True)
return result.stdout.splitlines()
def add_user_to_group(username, group):
cmd = f'Add-ADGroupMember -Identity "{group}" -Members "{username}"'
subprocess.run(["powershell", "-Command", cmd], check=True)
username = "example_user"
print(f"Groups for {username}:")
for group in get_user_groups(username):
print(group)
new_group = "IT_Department"
add_user_to_group(username, new_group)
print(f"Added {username} to {new_group}")
3. Automated System Health Check
Perform a comprehensive system health check:
import psutil
import platform
import subprocess
def system_health_check():
print(f"System: {platform.system()} {platform.version()}")
print(f"CPU Usage: {psutil.cpu_percent()}%")
print(f"Memory Usage: {psutil.virtual_memory().percent}%")
print(f"Disk Usage: {psutil.disk_usage('/').percent}%")
if platform.system() == "Windows":
subprocess.run(["powershell", "Get-WmiObject", "Win32_LogicalDisk"], check=True)
elif platform.system() == "Linux":
subprocess.run(["df", "-h"], check=True)
system_health_check()
Ready to Level Up Your IT Skills?
These Python scripts are just the beginning. Dive deeper into Python for IT and unlock your full potential!
Further Learning
Explore these advanced topics to further enhance your Python skills in IT:
Join Our Community!
π Get exclusive insights and the latest IT tools and scripts, straight to your inbox.
π We respect your privacy. Unsubscribe at any time.